Controlled flight advanced use is described on this page.
The capabilities and features can be expanded with added components. These can be written by the user or by using provided components. The components can also be studied to use as templates for new functionality.
Typically additional functionality is added by creating components. These components can react to events from the MissileSupervisor or by reading and writing to MissileSupervisor class members (see Script Reference).
Tracking and Launch sequence
Note that the tracking and launch sequence can only be done once for each MissileSupervisor. This means that once the object is launched it can not go back to tracking.
Copying the component values to a new object is the way to restart the sequence art the moment. If there is need for restarting, it can be added to Controlled Flight, request here.
2D
The use of the algorithms in 2D is easiest accomplished by using Constraints – Freeze Position in the Rigidbody.
Advanced aerodynamic drag
The drag modeled in MissileSupervisor is simplified. Among other simplifications the drag coefficient, Cd, is constant for each stage. This is typically not true when the speed changes, but should be enough for most use cases.
The component Advanced Drag provided with controlled flight changes Cd as a function of speed
Just add the Advanced Drag component to the object that has the MissileSupervisor and it will change Cd for the drag model.
This can be used to change drag for subsonic versus supersonic flight. Or add more drag in transsonic speeds.
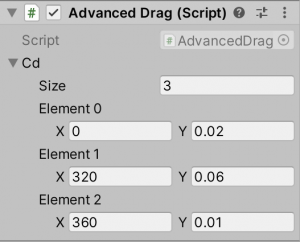
Using the values above simulates varying Cd for sub-, trans- and supersonic speeds. The transsonic range is assumed to be in 320-360 m/s for visualization, the range is typically smaller.
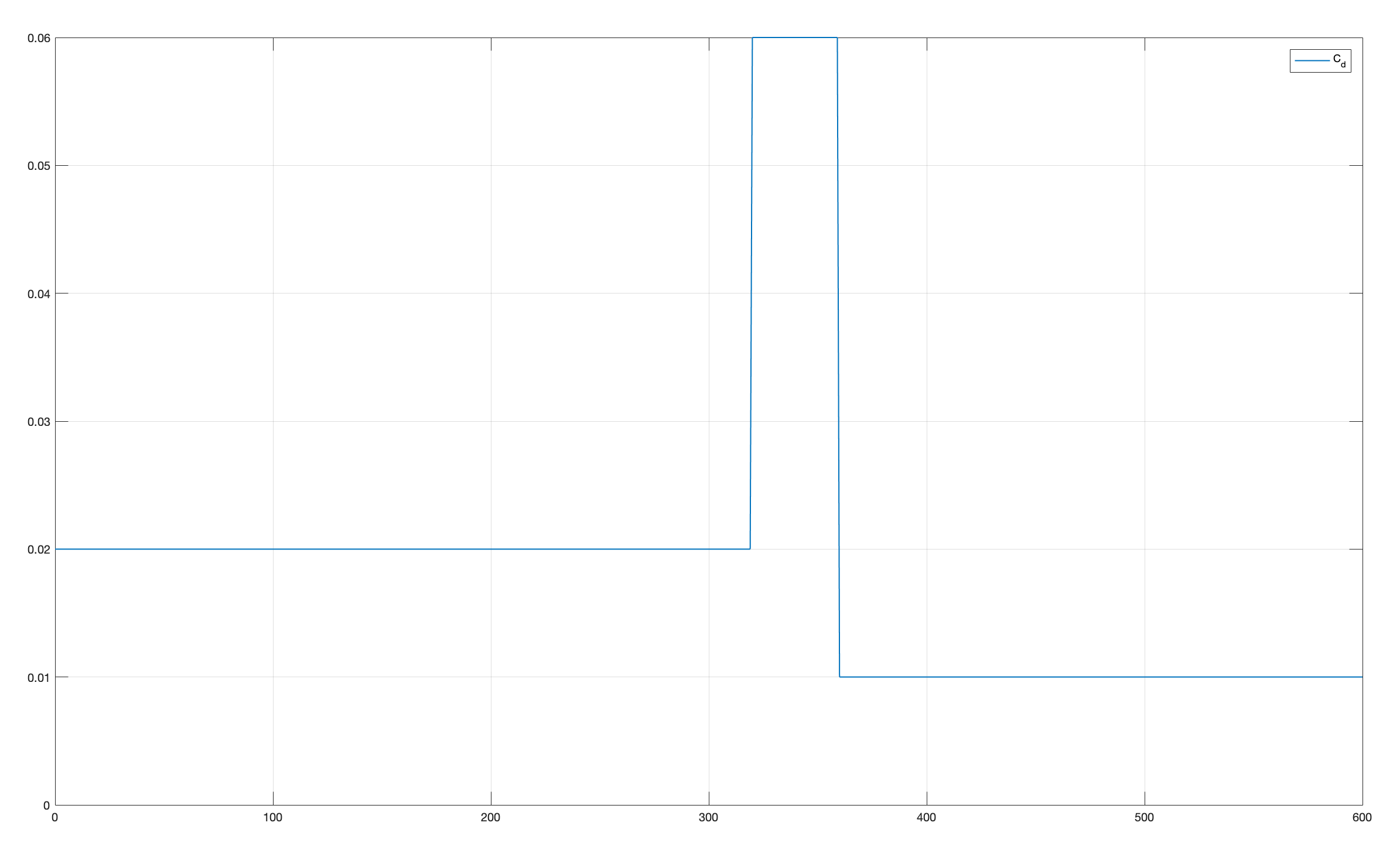
See code for the component for details, and to see how easy it is to add functionality. With small changes the drag can be further detailed, for example to take into account maneuvering.
Advanced maneuver limitation
The maneuver limitation in MissileSupervisor is simplified, but probably enough for most applications. However, the limitation is typically not constant. It typically depends on many factors, but mostly speed.
The component AdvancedManeouverLimitiation adds maneuver limitation as a function of speed. Just add the component and input limitations as a function off speed (sorted by speed).
The settings in the example gives the following limitations:
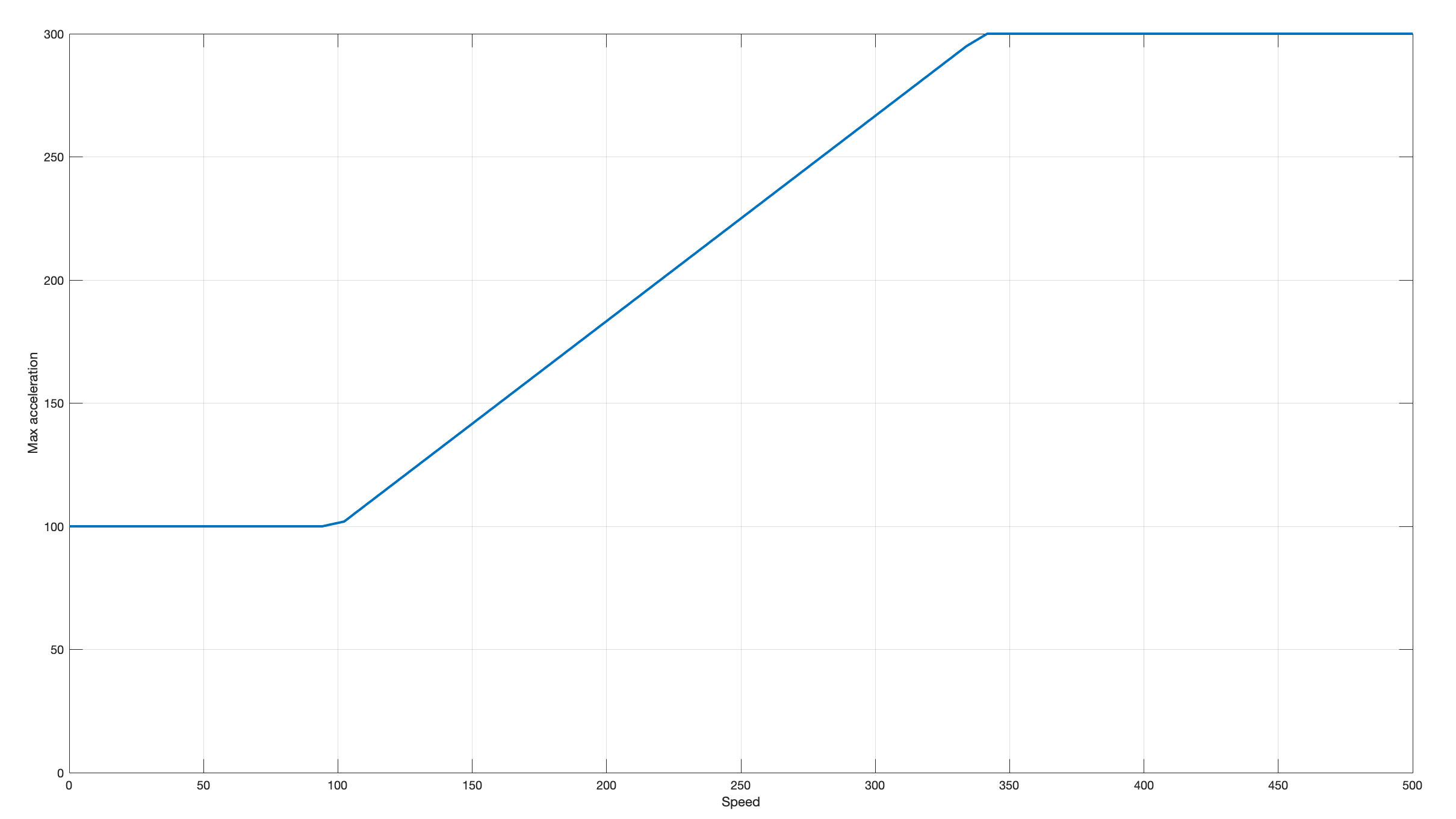
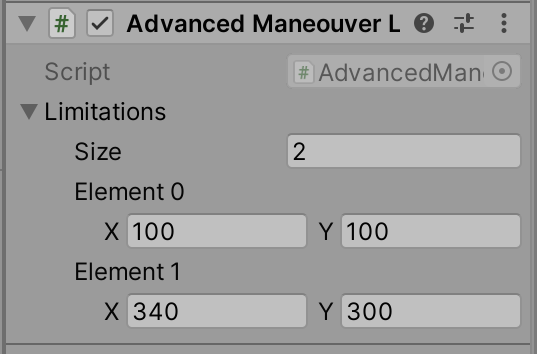
Change Speed at waypoint
It is simple to add a speed change when changing waypoints. Its can be done by listening to the Next Waypoint event and changing motor stage (or the settings of the motor stage) at invocation.
The code below for a new component will create waypoint dependent speed for the missile. It works by setting the speed value of a Constant speed motor stage at specified waypoint changes. It also have a default speed
This works under some assumptions: the motor stage will not change, the stage type is Constant Speed. These assumptions are done to simplify the code, but with some additions more flexibility can be added.
The settings above are: speed after waypoint 0=8 m/s and waypoint 2=12 m/s. all other waypoints uses default speed 4 m/s. For 5 waypoints this results in:
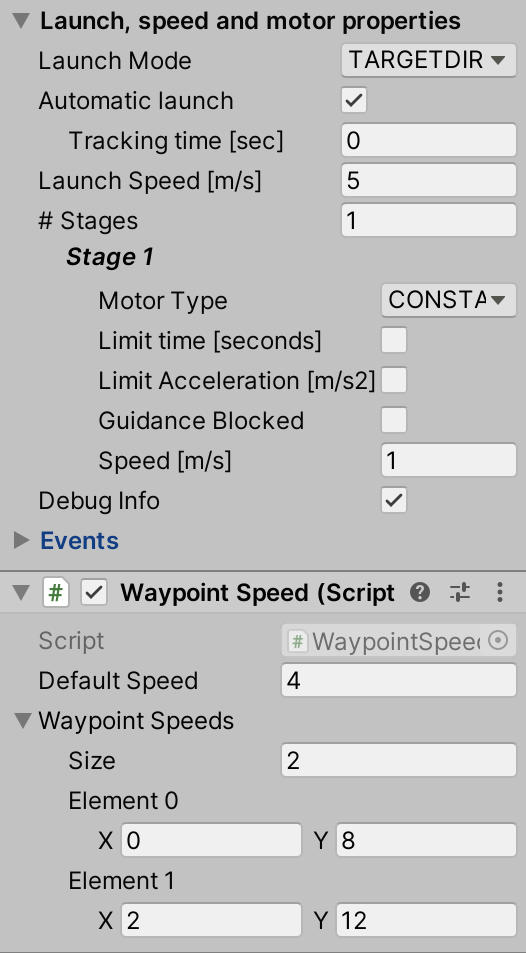
using System.Collections.Generic;
using UnityEngine;
[RequireComponent(typeof(MissileSupervisor))]
public class WaypointSpeed : MonoBehaviour
{
[Tooltip("Default speed used when waypoint not found in list")]
[SerializeField] public float m_defaultSpeed = default;
[Tooltip("Speeds (y) for waypoints (x)")]
[SerializeField] public List<Vector2> m_waypointSpeeds = new List<Vector2>();
private MissileSupervisor m_missileSupervisor = null;
private void Start()
{
m_missileSupervisor = GetComponent<MissileSupervisor>();
m_missileSupervisor.m_eventNextWayPoint.AddListener(ChangeSpeed);//React to waypoint change
}
private void ChangeSpeed(int waypoint)
{
float wantedSpeed;
//Find the relevant speed. Note that x is a float but is rounded to an int since it is a waypoint index
int speedWantedIdx = m_waypointSpeeds.FindIndex(item => Mathf.RoundToInt(item.x) == waypoint);
if (speedWantedIdx != -1)
wantedSpeed = m_waypointSpeeds[speedWantedIdx].y;
else
wantedSpeed = m_defaultSpeed;
ThrustControl.MotorSettings stage = m_missileSupervisor.m_motorStages[m_missileSupervisor.m_currentMotorStage];
stage.m_speed = wantedSpeed;
}
}
Flock of birds
Controlled flight can be used to emulate many types of objects. The included component Flock is an example that examples the simplicity of how a flock of birds can be spawned and moved. See code in Flock.cs and example scene BirdScene.
The result can be seen in the video below.