A reference collection of all parts of Controlled flight as well as some detailed information not available on other pages.
The main script/component to interact with is MissileSupervisor.
Every class specific for Controlled Flight is under the namespace SparseDesign.ControlledFlight.
Classes that are not specific to Controlled Flight, i.e. general accessories, are under namespace SparseDesign.
Main classes:
- MissileSupervisor
- MissileSupervisorInspector
- MissileGuidance
- ProportionalNavigation
- AugumentedProportionalNavigation
- CLOS
- ThrustControl
- ConstantSpeed
- NoThrust
- ConstantSpeed
- ConstantThrust
The custom Unity Editor seen in the Inspector is implemented in the class MissileSupervisorInspector.
Naming Conventions
Class names and method names uses Pascal Casing:
MissileSupervisor
missileSupervisor.Launch()
Variables (including member variables) uses Camel Casing. Class member variables have the prefix “m_”:
pathCount
missileSupervisor.m_autoLaunch
MissileSupervisor class
MissileSupervisor is the main class handling all movement of the object. The structure of member variables:
- MissileSupervisor
- m_guidanceSettings – Used to instantiate on MissileGuidance.
- m_targetType
- m_guidanceType
- etc…
- m_motorStages – Used to instantiate a ThrustControl for each motor stage.
- m_motorType
- m_speed
- etc…
- (public variables)
- m_guidanceSettings – Used to instantiate on MissileGuidance.
Each variable accessible in the Unity Editor have a tooltip including the variable name. It is indicated as, for example, [m_limitFlightTime], [m_guidanceSettings.m_targetType] or [m_motorStages[idx].m_motorType]. These variables are the accessed from scripts as:
missileSupervisor.m_limitFlightTime
missileSupervisor.m_guidanceSettings.m_targetType
missileSupervisor.m_motorStages[0].m_motorType
where missileSupervisor is an instance of the Missilesupervisor class/component. Many, but not all, variables can be changed after track and/or launch and dynamically affect the behavior. For example, guidance method can not change after launch.
Note that most variables are not sanity checked if changed outside the Unity Editor.
Method | Description |
---|---|
SetStageNo(int stage) | Sets the motor stage to use. Zero-based numbering. 0: first stage. Stages do not have to move used in order. |
Launch() Launch(bool forceLaunch) | Launches the missile if possible. Launch(true) launches (almost) immediately even if launch sequence has not been started. Launch(false) is identical to Launch(). |
TrackTarget() | Starts tracking. Will not automatically launch, see Launch(). |
StartLaunchSequence() | Starts launch sequence. Tracks target for some time (m_trackingDelay) and launches automatically. |
MissileGuidance class
MissileGuidance is the abstract class for the subclasses ProportionalNavigation, AugumentedProportionalNavigation (subclass to ProportionalNavigation) and CLOS. Instances of one of these classes do the guidance for the object.
Instantiate with:
public static MissileGuidance.GetNewInstance(GameObject missile, GuidanceSettings settings)
missile is the object to control with properties of settings. Note that different guidance types methods can be implemented with the same class. For examples Line-Of-Sight guidance and Constant Bearing both uses the CLOS class. See MissileGuidance.GetNewInstance().
Most of the behavior of the class is described in Target guidance and Path Following.
Variable | Description |
---|---|
m_currentCommand | Current commanded acceleration vector [Vector3, m/s² x 3]. Can be used by other scripts/classes to access the current commanded acceleration. Possible uses are: simulating rudder movements or other visual indication on the current maneuver. |
ThrustControl class
ThrustControl is the abstract class for the subclasses ConstantSpeed, NoThrust, ConstantSpeed and ConstantThrust. Instances of one of these classes controlled the speed of the object.
Instantiate with:
public static ThrustControl GetNewInstance(GameObject missile, MotorSettings settings)
missile is the object to control with properties of settings. Note that different speed control types can be implemented with the same class. See MissileGuidance.GetNewInstance().
Most of the behavior of the class can be found in Launch and speed control.
Member variables in ThrustControl:
Variable | Description |
---|---|
ThrustControl.m_rho | Density of air [float, kg/m³]. Used in drag calculations. Static variable shared by all scripts. |
Coordinate Systems
The object is moved in the standard Unity coordinate systems. This means that forward is the positive z-dir and up is positive y-direction.
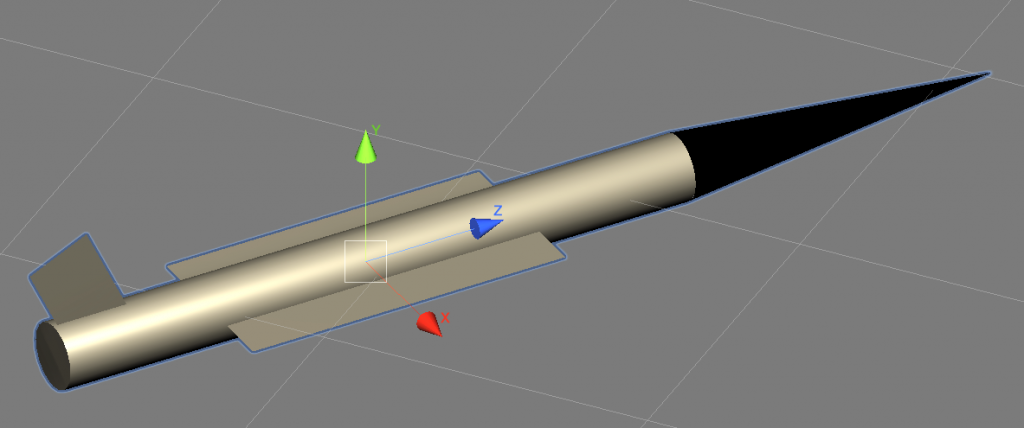